11.2. While loops#
For loops are useful for when we know how many times we want to repeat a set of commands. Sometimes we might not know this and would like to loop until a certain condition is met. This is where while loops come in. The MATLAB syntax for a while loop is
while condition
% commands
end
The lines that are betwee the while loop declaration and end
will be executed while the condition
returns a true result. For example, to print the numbers 0 to 4 as we did with a for loop add the following code into your program.
% While loops
i = 1;
fprintf("\n")
while i < 6
fprintf("%d\n", i)
i = i + 1;
end
With while loops we don’t have a loop variable so we have defined the variable i
and set it equal to 1. Then we have declared a while loop to loop whilst i
is less than 6. Inside the while loop we use a fprintf()
command to print the current value of i
before incrementing it by 1. Run your program and you should see the following added to the console output.
1
2
3
4
5
Warning
A common programming error when dealing with while loops is forgetting to update the while loop condition. This can result in the condition always being true and the commands in the while loop being executed forever. This is known as an infinite loop.
To demonstrate this, delete or comment out the i = i + 1
command in the while loop you have just written and rerun the program. You should set lots of 1s being printed to the console since the i
variable is always 1 which is of course less than 5. To stop the program click on the stop icon \(\blacksquare\).
11.2.1. Approximating the Golden ratio using Fibonacci numbers#
The golden ratio is the ratio between two numbers, \(a\) and \(b\), where \(a+b\) is to \(b\) as \(b\) is to \(a\) and is denoted by \(\varphi\). The actual value of \(\varphi\) is
A property of the Fibonacci sequence is that the ratio between two successive numbers in the sequence, \(F_{n} / F_{n-1}\), converges to \(\varphi\) as \(n\) gets large (note that this does not work for the first two numbers since \(F_0 = 0\) and we cannot divide by zero), for example
Lets use a while loop to calculate the approximation of \(\varphi\) until two successive approximations are within \(10^{-8}\) of each other. Enter the following code into your program.
% Approximating the golden ratio
a = 1;
b = 1;
new_phi = 1;
old_phi = 0;
fprintf("\n%0.6f", new_phi)
while abs(new_phi - old_phi) > 1e-6
c = a + b;
a = b;
b = c;
old_phi = new_phi;
new_phi = b / a;
fprintf("%0.6f\n", new_phi)
end
Here we start by declaring a
and b
to be the second and third Fibonacci numbers (we can’t use the first Fibonacci number as that would mean dividing by zero) and declare two variables new_phi
and old_phi
which contain the successive approximations of \(\varphi\). We then use a while loop to repeat calculations until the absolute difference between new_phi
and old_phi
is less than \(10^{-6}\). Inside the while loop we calculate the next Fibonacci number, update the approximations of \(\varphi\) and print out the current approximation.
Run your program and you should see the following added to the console.
1.000000
2.000000
1.500000
1.666667
1.600000
1.625000
1.615385
1.619048
1.617647
1.618182
1.617978
1.618056
1.618026
1.618037
1.618033
1.618034
1.618034
So our program finishes with an approximation of \(\varphi \approx 1.618034\) which agrees with the actual value correct to 6 decimal places. Using while loops to iterate a solution until convergence is a common technique used in numerical methods.
11.2.2. Exercises#
Exercise 11.4
Use a while loop to print “hello again” ten times.
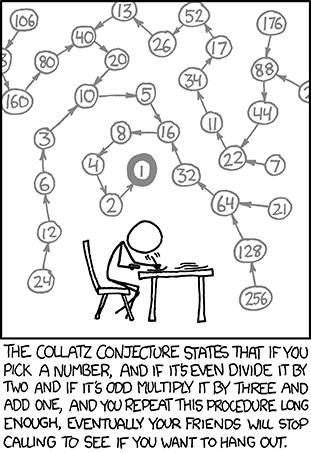
Fig. 11.1 https://xkcd.com/710#
Exercise 11.5
The Collatz conjecture states that the series generated by the following rules will eventually reach 1.
For example, for \(x_0 = 10\) the sequence is
and it took 6 steps to reach 1. Write a program that uses a while loop to print the numbers in the Collatz sequence for a given starting number. How many steps does it take for a starting number of 100 to reach 1?